F:\work> mvn archetype:generate -DarchetypeCatalog=http://kallisti.eoti.org:8081/content/repositories/snapshots/archetype-catalog.xml
Choose the galatea-archetype plugin
groupId: org.eoti.android
artifactId: TabTest
version: 1.0-SNAPSHOT
package: org.eoti.android
Make sure the emulator is running...
F:\work> cd TabTest
F:\work\TabTest> mvn clean install
Replace the contents of your src\main\android\res\layout\main.xml:
<?xml version="1.0" encoding="utf-8"?>
<TabHost xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@+id/my_tabhost"
android:layout_width="fill_parent"
android:layout_height="fill_parent">
<TabWidget
android:id="@android:id/tabs"
android:layout_width="fill_parent"
android:layout_height="65px"/>
<FrameLayout
android:id="@android:id/tabcontent"
android:layout_width="fill_parent"
android:layout_height="200px"
android:paddingTop="65px">
<LinearLayout
android:id="@+id/content1"
android:orientation="vertical"
android:layout_width="fill_parent"
android:layout_height="fill_parent">
<TextView
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:text="@string/weather"
/>
</LinearLayout>
<LinearLayout
android:id="@+id/content2"
android:orientation="vertical"
android:layout_width="fill_parent"
android:layout_height="fill_parent">
<TextView
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:text="@string/faces"
/>
</LinearLayout>
</FrameLayout>
</TabHost>
Replace the contents of your src\main\android\res\values\strings.xml:
<?xml version="1.0" encoding="utf-8"?>
<resources>
<string name="app_name">TabTest</string>
<string name="weather">This is the weather tab</string>
<string name="faces">This is the faces tab</string>
</resources>
Insert this into the end of your onCreate method in your activity (src\main\java\org\eoti\android\TabTest.java in my case):
TabHost tabs = (TabHost) this.findViewById(R.id.my_tabhost);
tabs.setup();
TabHost.TabSpec spec1 = tabs.newTabSpec("First tab");
spec1.setIndicator("Weather");
spec1.setContent(R.id.content1);
tabs.addTab(spec1);
TabHost.TabSpec spec2 = tabs.newTabSpec("Second tab");
spec2.setIndicator("Face");
spec2.setContent(R.id.content2);
tabs.addTab(spec2);
tabs.setCurrentTabByTag("First tab");
Redeploy (mvn clean install) and you will have some basic text-based tabs...
But, maybe we want some graphics too... This tutorial says:
You need an icon for each of your tabs. For each icon, you should create two versions: one for when the tab is selected and one for when it is unselected. The general design recommendation is for the selected icon to be a dark color (grey), and the unselected icon to be a light color (white).But I wanted to be a little more creative, so I grabbed a couple icons from the Tango project [note: had to replace '-' in filenames with '_']:
Download these images into src\main\android\res\drawable\




Create face.xml into src\main\android\res\drawable:
<?xml version="1.0" encoding="utf-8"?>
<selector xmlns:android="http://schemas.android.com/apk/res/android">
<item android:drawable="@drawable/face-devilish" android:state_selected="true" />
<item android:drawable="@drawable/face-angel" />
</selector>
Create weather.xml into src\main\android\res\drawable:
<?xml version="1.0" encoding="utf-8"?>
<selector xmlns:android="http://schemas.android.com/apk/res/android">
<item android:drawable="@drawable/weather-showers-scattered" android:state_selected="true" />
<item android:drawable="@drawable/weather-snow" />
</selector>
In your activity, replace this line:
spec1.setIndicator("Weather");with:
spec1.setIndicator("Weather", this.getResources().getDrawable(R.drawable.weather));
And replace this:
spec2.setIndicator("Face");with:
spec2.setIndicator("Face", this.getResources().getDrawable(R.drawable.face));
While we are at it, open your AndroidManifest.xml and replace this line:
<activity android:name=".TabTestActivity">with:
<activity android:name=".TabTestActivity" android:theme="@android:style/Theme.NoTitleBar">
Redeploy (mvn clean install) and you will have nice icons on your tabs...
End result should look like these:
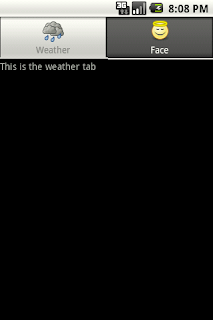
It is also possible to have the tabs load different Views or Activities by having the activity extend TabActivity. Maybe I'll do another tutorial for that.
what i want to implement is, how i can change the default background grey color of all tabs to another color. The tabs contain only text but not images or icons. Its urgent.can any one help me on this.
ReplyDeleteHi,
ReplyDeleteThanks for Ur Artical.
I have one doubt, how to display the tabs in the bottom of the Android device.Would you reply for this doubt ASAP.
@bob -- take a look at http://groups.google.com/group/android-developers/browse_thread/thread/46c11c89fd7e3e17/e3c73b966fa4243b?lnk=raot
ReplyDelete@cse -- take a look at http://stackoverflow.com/questions/2395661/android-tabs-at-the-bottom
thanks for ur reply.But I tried this its not working for me let me know is any other way?
ReplyDeleteThanks,
Laskhmanan
@cse unfortunately, I don't know. That should have worked.
ReplyDeleteI followed your code and description, but I couldn't make images used for tabs visible. Can you please let me know the reason why this is happening?
ReplyDelete@Laskhmanan - I'd need more info to be able to help with that. What *does* it do? What version of Android? Emulator or hardware? Have you checked Logcat?
ReplyDelete